How I Made Destructible UI for Blasteroids
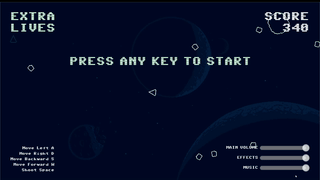
Building destructible UI with gameplay effects in Godot is basically the same as building other destructible objects with gameplay effects. There are lots of ways to do it, and what I explain here is just how I did it for Blasteroids.
I started with a Label as most of the UI is text. I added a standard collision setup with Area2D and CollisionShape2D with a rectangle.
The Label is the root node for ease of customization when assembling into bigger UI elements.
The collision shape had to stay in sync with the label’s size changes. The resized
signal works for that.
func _on_label_resized() -> void:
# Move area2d to center of label
$Area2D.position = size / 2
# Make rectangle shape 2d of collision the same size as label
$Area2D/CollisionShape2D.shape.size = size
Hits and damage are handled like any other object, e.g. area_entered
and/or body_entered
signals and queue_free()
to remove the destroyed nodes. Don’t forget the sfx & vfx juice!
Along with CollisionLabel, a CollisionTextureRect was created for the extra lives markers and CollisionHSlider for the volume controls. Those three types handle the entire UI.
To get the gameplay consequences of destroying UI elements, I used the tree_exiting
signals of the controls and some flag variables. For example, when building the actions UI labels:
$ActionLabelMoveRight.tree_exiting.connect(
func(): Globals.can_move_right = false
)
Then in the player input code:
if Input.is_action_pressed("move_right") and Globals.can_move_right:
# Turn right code
We were reminded by our playtesters that when changing scenes, even with get_tree().reload_current_scene()
all nodes leave the tree. So, after the first game over all controls stopped working whether you blew them up or not! I fixed that by reseting the Globals on game start. Always allow time for playtester feedback!!
There was some debate about whether destroying the UI elements should have the same effect (stop the input) or something more chaotic, like always on or intermittently on. For the jam, we guessed that the better player experience would be a predictable “stops working” state. I look forward to your comments about how wrong we were!
Along with more chaos and explosions, what do you wish was in the game?
Blasteroids
Shoot all the things in this classic arcade game homage
Status | In development |
Authors | hatmix, Muskets, fradono |
Genre | Action |
Tags | Arcade, Destruction, Space |
Languages | English |
Leave a comment
Log in with itch.io to leave a comment.